Friday, 29 July 2011
Implement AndroidManifest.xml
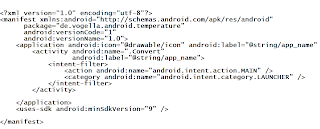
An Android application is described the file "AndroidManifest.xml". This file must declare all activities, services, broadcast receivers and content provider of the application. It must also contain the required permissions for the application. For example if the application requires network access it must be specified here. "AndroidManifest.xml" can be thought as the deployment descriptor for an Android application.
The "package" attribute defines the base package for the following Java elements. It also must be unique as the Android Marketplace only allows application for a specfic package once. Therefore a good habit is to use your reverse domain name as a package to avoid collisions with other developers.
"android:versionName" and "android:versionCode" specify the version of your application. "versionName" is what the user sees and can be any string. "versionCode" must be an integer and the Android Market uses this to determine if you provided a newer version to trigger the update on devices which have your application installed. You typically start with "1" and increase this value by one if you roll-out a new version of your application.
"activity" defines an activity in this example pointing to the class "de.vogella.android.temperature.Convert". For this class an intent filter is registered which defines that this activity is started once the application starts (action android:name="android.intent.action.MAIN"). The category definition (category android:name="android.intent.category.LAUNCHER" ) defines that this application is added to the application directory on the Android device. The @ values refer to resource files which contain the actual values. This makes it easy to provide different resources, e.g. strings, colors, icons, for different devices and makes it easy to translate applications.
The "uses-sdk" part of the "AndroidManifest.xml" defines the minimal SDK version your application is valid for. This will prevent your application being installed on devices with older SDK versions.
Thursday, 28 July 2011
Main component of android application
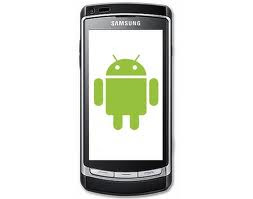
�� Activities: The building block of the user interface is the activity. You
can think of an activity as being the Android analogue for the window
or dialog box in a desktop application. While it is possible for activities
to not have a user interface, most likely your “headless” code will be
packaged in the form of content providers or services.
�� Content providers: Content providers provide a level of abstraction for
any data stored on the device that is accessible by multiple
applications. The Android development model encourages you to
make your own data available to other applications, as well as your
own. Building a content provider lets you do that, while maintaining
complete control over how your data is accessed.
�� Services: Activities and content providers are short-lived and can be
shut down at any time. Services, on the other hand, are designed to
keep running, if needed, independent of any activity. You might use a
service for checking for updates to an RSS feed or to play back music
even if the controlling activity is no longer operating.
�� Intents: Intents are system messages, running around the inside of the
device, notifying applications of various events, from hardware state
changes (e.g., an SD card was inserted), to incoming data (e.g., an
SMS message arrived), to application events (e.g., your activity was
launched from the device’s main menu). Not only can you respond to
intents, but you can create your own to launch other activities or to let
you know when specific situations arise (e.g., raise such-and-so intent
when the user gets within 100 meters of this-and-such location).
Wednesday, 27 July 2011
SEO
Welcome to SEO.com: a search marketing firm with the knowledge, skills, and a proven process that consistently delivers results. Results that deliver a significant increase in traffic and a Big ROI.
Here’s how we do it: We form a strategic Internet marketing plan around search engine optimization (SEO), pay per click advertising, social media marketing, conversion optimization and search-optimized Web design. Then, we tenaciously go to work so you can quickly dominate your online competition.
So, start dominating today. Request a proposal from a top SEO company and let’s set up a chance for high search engine rankings, more customers and a big return on your investment.
What is SEO?
Search engine optimization is a method of getting your website to rank higher in search engines—such as Google, Yahoo or Bing. A search engine optimization campaign pairs on-site optimization with off-site tactics, which means you make changes to your site itself while building a portfolio of natural looking back links to increase your organic rankings. When Internet users search for your products or services, your website needs to be the first one they find. SEO helps the search engines recognize your relevance to specific keywords that people search for online. The search engine optimization process includes researching keywords, creating content, building links and making sure your website is visible in the search engines.
Thursday, 21 July 2011
Configuring Your Development Environment
Java development.The software is available online for download at no cost.Android applications
can be developed on Windows, Macintosh, or Linux systems.
To develop Android applications, you need to have the following software installed on
your computer:
1) The Java Development Kit (JDK) Version 5 or 6, available for download at http://java.sun.com/javase/downloads/index.jsp.
2)A compatible Java IDE such as Eclipse along with its JDT plug-in, available for download at http://www.eclipse.org/downloads/.
3) The Android SDK, tools and documentation, available for download at http://developer.android.com/sdk/index.html.
4) The Android Development Tools (ADT) plug-in for Eclipse, available for download through the Eclipse software update mechanism. For instructions on how to install this plug-in, see http://developer.android.com/sdk/eclipse-adt.html.Although this tool is optional for development,we highly recommend it and will use
its features frequently throughout this book.
Android Platform Differences
1) Complete: The designers took a comprehensive approach when they developed the
Android platform.They began with a secure operating system and built a robust software
framework on top that allows for rich application development opportunities.
2) Open: The Android platform is provided through open source licensing. Developers
have unprecedented access to the handset features when developing applications.
3) Free: Android applications are free to develop.There are no licensing or royalty fees
to develop on the platform. No required membership fees. No required testing fees.
No required signing or certification fees.Android applications can be distributed
and commercialized in a variety of ways.
Wednesday, 20 July 2011
jquery example
Simple Mistakes Android Developer
This post talks about some challenges that you may run into early in your foray into Android development. There isn’t really any sense of order to these; they’re more-or-less a brain dump of issues I’ve experienced or seen that take seconds to solve if you know what you’re doing or hours if you don’t. OMG! The emulator is slow! There are two things to note here: the default AVDs tend to have limited resources and the devices you’re targeting are likely running with a different chipset than the computer you’re developing on.
The first limitation can be easily overcome; the second is a much bigger issue, particularly with Android 3.x and attempting to develop for Honeycomb tablets without actually having a Xoom, Asus Eee Pad Transformer, etc.
Currently, it’s not very feasible to develop a 3.x app without a tablet. Back to that first limitation: When creating a new AVD, you can specify hardware settings, which can dramatically improve performance. The most important setting is the device RAM size; I tend to want the emulator to be as fast as possible, relying on on-device testing for a sense of “real-world speed,” so I usually give my phone emulators 512MB of RAM. That’s the equivalent of most good smartphones from 2010. The 1.x AVDs seem to run fine on 256MB, so you can potentially drop to that if your machine is limited (the Motorola Droid/Milestone had 256MB of RAM as well).
You can also try upping the cache partition size (128MB is a good start) and the max VM application heap size (48 is plenty) for a minor improvement. LinearLayout mistakes LinearLayout is a very useful and very simple ViewGroup. It essentially puts one View after another either horizontally or vertically, depending on the orientation you have set. Set an orientation! LinearLayout defaults to horizontal, but you should always manually specify an orientation. It’s very easy to throw two Views in there and not see one because the first View is set to match_parent for width.
That said, LinearLayout also processes layouts linearly, which is what you’d expect, but that means that setting a child View to match_parent can leave no room for subsequent Views. If you aren’t seeing one of your Views in a LinearLayout, verify you’ve set the orientation and that none of the Views are taking up all the available space. Within a LinearLayout, you should generally rely on layout_weight to “grow” the View.
Here’s an example: The heights are set to 0, but both child Views have weights. The first has a weight of 2 and the second has a weight of 3. Out of a total of 5, the first is taking 2 (40%) and the second gets 3 (60%), so their heights will actually use that portion of the parent View’s space (if that View has a 100px height, the first child will be 40px tall). The weights are relative, so you can set whatever values make sense for you.
In this example, the second layout could have had a set height and no weight and the first could have had a weight of 1, which would have made the first take up all space that the second didn’t use. Stupid Android! It keeps restarting my Activity! The default behavior when a configuration change happens (e.g., orientation change, sliding out the keyboard, etc.) is to finish the current Activity and restart it with the new configuration.
If you’re not getting any dynamic data, such as loading content from the web, this means you probably don’t have to do any work to support configuration changes; however, if you are loading other data, you need to pass it between configuration changes. Fortunately, there’s already a great post on Faster screen orientation changes. Read it and remember that you should not pass anything via the config change methods that is tied to the Activity or Context. Density Density should only be considered in terms of density not size. In other words, don’t decide that MDPI means 320px wide. It doesn’t.
It means that the device has around 160dpi like the G1 (320px wide) and the Xoom (1280px wide). Fortunately, you can combine multiple qualifiers for your folders to give precise control, so you can have a drawable-large-land-hdpi/ containing only drawables that are used on large HDPI devices that are in landscape orientation. One other important thing to note: Support for different screen sizes and densities came in Android 1.6. Android 1.5 devices are all MDPI devices, but they don’t know to look in drawable-mdpi/ because there were no different densities then.
So, if you’re supporting Android 1.5, your MDPI drawables should go in drawables/. If your minSdkVersion is 1.6 or above, put the MDPI drawables in drawable-mdpi/. What the heck is ListView doing? A full explanation of ListView would take quite a while, but here’s a quick summary: A ListView’s associated Adapter’s getView method is called for each child View it needs to construct to fill the ListView.
If you scroll down, the top View that leaves the screen is not garbage collected but is instead passed back to the getView method as the “convertView” (second param). Use this View! If you don’t, not only does it need to be garbage collected, you have to inflate new Views. You should also use the ViewHolder paradigm where you use a simple class to store View references to avoid constant findViewById() calls.
Here’s an example: @Override public View getView(int position, View convertView, ViewGroup parent) { final ViewHolder holder;
if (convertView == null) {
convertView = mInflater.inflate(R.layout.some_layout, null);
holder = new ViewHolder();
holder.thumbnail = (ImageView) convertView.findViewById(R.id.thumbnail);
holder.title = (TextView) convertView.findViewById(R.id.title);
convertView.setTag(holder);
}
else {
holder = (ViewHolder) convertView.getTag();
}
final MyObject obj = (MyObject) getItem(position);
holder.thumbnail.setImageDrawable(obj.getThumbnail());
holder.title.setText(Html.fromHtml(obj.getTitle()));
return convertView;
} /** * Static ViewHolder class for retaining View references*/
private static class ViewHolder {
ImageView thumbnail; TextView title;
}
This method is first checking if the convertView exists (when the ListView is first laid out, convertView will not exist). If it doesn’t exist, a new layout is inflated with the LayoutInflater. A ViewHolder object is instantiated and the View references are set. Then, the holder is assigned to the View with the setTag method (which essentially allows you to associate an arbitrary object with a View).
If the convertView already exists, all that has already been done, so you just have to call getTag() to pull that ViewHolder out. The “bulk” of the method is just grabbing MyObject (whatever object your Adapter is connecting to) and setting the thumbnail ImageView and the title TextView.
You could also make the ViewHolder fields final and set them in the constructor, but I tried to keep this example as simple as possible. This method no longer requires View inflation while scrolling nor does it require looking through the Views for specific IDs each time. This little bit of work can make a huge different on scrolling smoothness. One last thing about ListViews… never set the height of a ListView to wrap_content.
If you have all your data locally available, it might not seem so bad, but it becomes particularly troublesome when you don’t. Consider the above example but instead of the setImageDrawable call, it triggers an image download (though it’s better to have the Adapter handle that).
If you use wrap_content on your ListView, this is what happens: The first getView call is done, convertView is null, and position 0 is loaded. Now, position 1 is loaded, but it is passed the View you just generated for position 0 as its convertView. Then position 2 is loaded with that same View, and so on. This is done to lay out the ListView since it has to figure out how tall it should be and you didn’t explicitly tell it.
Once it has run through all of those positions, that View is passed back to position 0 for yet another getView call, and then position 1 and on are loaded with getView and no convertView. You’re going to end up seeing getView called two or three times as often as you would have expected.
Not only does that suck for performance, but you can get some really confusing issues (e.g., if you had been passing a reference to an ImageView with an image download request, that same ImageView could be tied to all of the download requests, causing it to flicker through the images as they return).
Saturday, 9 July 2011
JavaScripts Page Refresh/Reload
There are times when the web-author would like their web-page to automatically refresh (or reload) at specified intervals. This is often useful when the page contains time sensitive information.
We discuss three versions:
JavaScript Refresh - preferred method
JavaScript Button Refresh
meta-tag - provided for reference but not the preferred method.
JavaScript Refresh
The preferred page refresh/reload method uses a JavaScript technique that will replace the current page with each refresh in the visitor's page history.
This version uses an under-utilized method of dealing with cross browser and old version browser compatibility. It defines multiple JavaScript code blocks, where each JavaScript version block redefines the same function. Only the last version block that is supported by the browser will be used. So older browsers will use the "JavaScript" block, while later browsers will use either the "JavaScript1.1" or "JavaScript1.2" block depending upon the browser's capabilities. If the browser does not support JavaScript or has it disabled, the "noscript" block will be used.
We do use the "refresh" meta-tag as a backup method to the JavaScript.
See this script in action.
JavaScript Refresh Button
A similar JavaScript will refresh/reload the page when the visitor clicks on a link, on an image/button, or a form button. In similar fashion we use the technique that will replace the current page with each refresh in the visitor's page history.
See this script in action.
Refresh Link

"refresh" meta-tag
The biggest problem with the "refresh" meta-tag method of page-refresh is that it can add to the visitor's page-history on various versions of browsers. This means that for each automatic page refresh/reload your site visitor must select the browser's back button. This potentially can be a considerable bother to your visitors as well as a confusion.
The preferred refresh method is "JavaScript Refresh"
See this script in action.
-->
Want your business simplified
- Visibility into all areas of the company- “After implementing an ERP, we get consolidated daily reports of inventory and other departments” avers Mr. Saurabh Singh, IT Head, Anand NVH Products Pvt. Ltd. By having an ERP at your place, you can have a visibility of different functional areas of your company. “Through an ERP, a company can have a check on its resources” adds Handa.
- Quality management for enhanced productivity- Productivity will increase if the management of the company is done effectively. An ERP helps you do that.
- Provides transparency and makes data available across various functions- An ERP gives accurate information at the right time to the right people for effective decision making. It provides you with a single access point for all data of the company. “ERP helps in getting compiled reports and ensures smooth workflow” maintains Handa.
- Helps to reduce operating costs and enhances efficiencies- ERP helps to improve co-ordination and increases the efficiency by reducing the operating costs like inventory, supply chain, production, marketing and help desk support costs.
- Planning for future- Through an ERP, you can analyse your current situation and plan strategically for future.
- Vishesh Infotech: Offers Business Soft a distribution and finance package, and Business Pro, which is meant for companies operating in the manufacturing segment.
- Eastern Software Systems (ESS): Their product, ‘ebizframe’ based on Oracle technology is built specially for small and medium businesses.
- Exainfotech Pvt. Ltd. also provides ERP for small and medium level businesses.
- Impact ERP software solution India- provides ERP for manufacturer or traders from small businesses to mid size enterprises.
- Sofgen computer consultants Pvt. Ltd. provides customised ERP solutions to various private and public sectors.
- Mind Infotech’s product, eMPro is specially built for the process industry.
A marketing plan
A marketing plan serves as a roadmap for your marketing activities to reachyour goals. To lay out an effective plan, begin with understanding the marketand then plan out the tactics and strategies required to reach out to yourultimate customers. To put it succinctly, a marketing plan should cover thestrategic four 4Ps- Product, Price, Place and Promotion.
Chalk out your marketing plan by following a simple step by step procedureas under.
1. Identify Your Business Goal
The first step is to spell out your business goal in your marketing plan.Say if you are company producing shoes, then your business goal could be like,“producing 1000 units per month” or “diversifying you business to othersectors”. The objective should be clear, feasible and realistic and notexaggerated or overambitious.
2. Define Your Target Customer
The marketing plan should have a clear picture of the target customer. ‘Arethey individuals or a company’. Lookout for some clearly identifiable and uniquecharacteristic features. Do they fall into a certain age group, income group orgeographic location? How do they buy your type of products or services? Howoften do they buy them? What features are they particular about?
3. Leverage Your Product’s USP
You do not market the product or the service, you market the benefitsattached to them. Describe these here. Do mention the distinctive and uniquefeatures (USP) that your product has which sets it apart from your competitor’sproduct. While thinking of the USPs, you must keep in mind to market thestrengths of your product and minimise your product’s weakness and at the sametime develop strategies to take advantage of your competitor’s weakness.
4. Position Your Product
It is very important to understand as to how you want your product to beperceived or positioned in the minds of your end customers and yourcompetitors. Base your positioning on the benefit your product provides, yourcustomers and the positioning of your competitors. Ask yourself- Do you want topromote your product as one having the best quality with little reference toprice or do you want to promote your product as the cheapest in the industry?
5. Decide Your Marketing Tactics
Here, you should describe the marketing tactics that you intend to use toreach out to your customers. Take for example advertising (print, radio,television), public relations, sales promotion, Classified ads, Contests,Public relations, Free samples and many others “The means of marketing should dependon the type of product that you are selling. Online or web marketing is bestsuited for us” says Mr. Vishal Bisht, CEO, Marksman Technologies, a companyinto E-learning and IT services.
6. Budget Your Marketing Expenditure
Here you need to mention the amount of money that you plan to invest in yourmarketing activities. You can break it into monthly, quarterly or annual basis.The amount that you have allocated for your marketing activities will depend onyour type of business and the goals that you have set. Keep track of howeffective a marketing tactic is as you need to get maximum returns on yourinvestment.